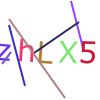
在使用React做前端,用户注册页面因为要短信验证,短信服务商要求加人机验证,于是我找到了 react-captcha-generator。But……
感谢作者,非常好用,但……短信服务商认为这个生成的图片太简单,“很简单,再加上几条横线就行……”。但怎么加横线呢?先找别的人机验证轮子,实在没有更合适的。自己从头做一个吧,又实在值不当的。
能不能在react-captcha-generator基础上修改呢?打开源码研究,谷歌搜如何在svg上加直线,还真弄成了。以下是控件代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89
| import React, { Component } from 'react';
class ReactCaptchaGenerator extends Component {
constructor(props) { super(props) this.state = { height: 100, width: 100, textColor: false, fontFamily: 'Verdana', fontSize: '30', paddingLeft: '20', paddingTop: '60', lenght: '5', background: 'none' } this.setData = this.setData.bind(this) }
componentWillMount() { this.setData() }
setData() { this.setState({ height: this.props.height ? this.props.height : 100, width: this.props.width ? this.props.width : 100, textColor: this.props.textColor ? this.props.textColor : false, fontFamily: this.props.fontFamily ? this.props.fontFamily : 'Verdana', fontSize: this.props.fontSize ? this.props.fontSize : '30', paddingLeft: this.props.paddingLeft ? this.props.paddingLeft : '20', paddingTop: this.props.paddingTop ? this.props.paddingTop : '60', lenght: this.props.lenght ? this.props.lenght : '5', background: this.props.background ? this.props.background : 'none', })
this.text = [] this.originText = [] this.possible = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789"; for (var i = 0; i < this.state.lenght; i++) { let char = this.possible.charAt(Math.floor(Math.random() * this.possible.length)) this.text.push( `<text font-family="${this.state.fontFamily}" font-size="${this.state.fontSize}" x="${this.state.paddingLeft * i}" y="${this.state.paddingTop}" fill="${ this.props.textColor ? this.props.textColor : "#" + ((1 << 24) * Math.random() | 0).toString(16)}" transform="rotate(${Math.random() * (5 - 0) + 0})" >${char}</text>` ) this.originText.push( char ) let x1 = Math.floor(Math.random() * this.state.width) let x2 = Math.floor(Math.random() * this.state.width) let y1 = Math.floor(Math.random() * this.state.height) let y2= Math.floor(Math.random() * this.state.height) this.text.push( `<line x1="${x1}" y1="${y1}" x2="${x2}" y2="${y2}" style="stroke:rgb(255,0,0);stroke-width:2" />` ) } this.props.result(this.originText.join(''))
}
render() {
return ( <img style={{ background: this.state.background }} src={ 'data:image/svg+xml;base64,' + btoa('<svg ' + 'xmlns="http://www.w3.org/2000/svg" ' + 'height="' + this.state.height + '" ' + 'width="' + this.state.width + '">' + this.text.join() + '</svg>') } alt="" /> ); } }
export default ReactCaptchaGenerator;
|
以下是调用控件的代码:
1 2 3 4 5 6 7 8 9 10 11 12 13
| import ReactCaptchaGenerator from './Captcha';
class App extends Component { constructor(props): super(props); this.state = {captcha:'',} ... onCaptchaMade = (r) => { this.setState({captcha:r,}); } ... <ReactCaptchaGenerator result={this.onCaptchaMade} /> ...
|
原来的验证图片:
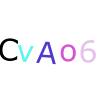
修改之后的验证图片:
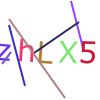
再次感谢原控件作者Vetal StekolschikovV。